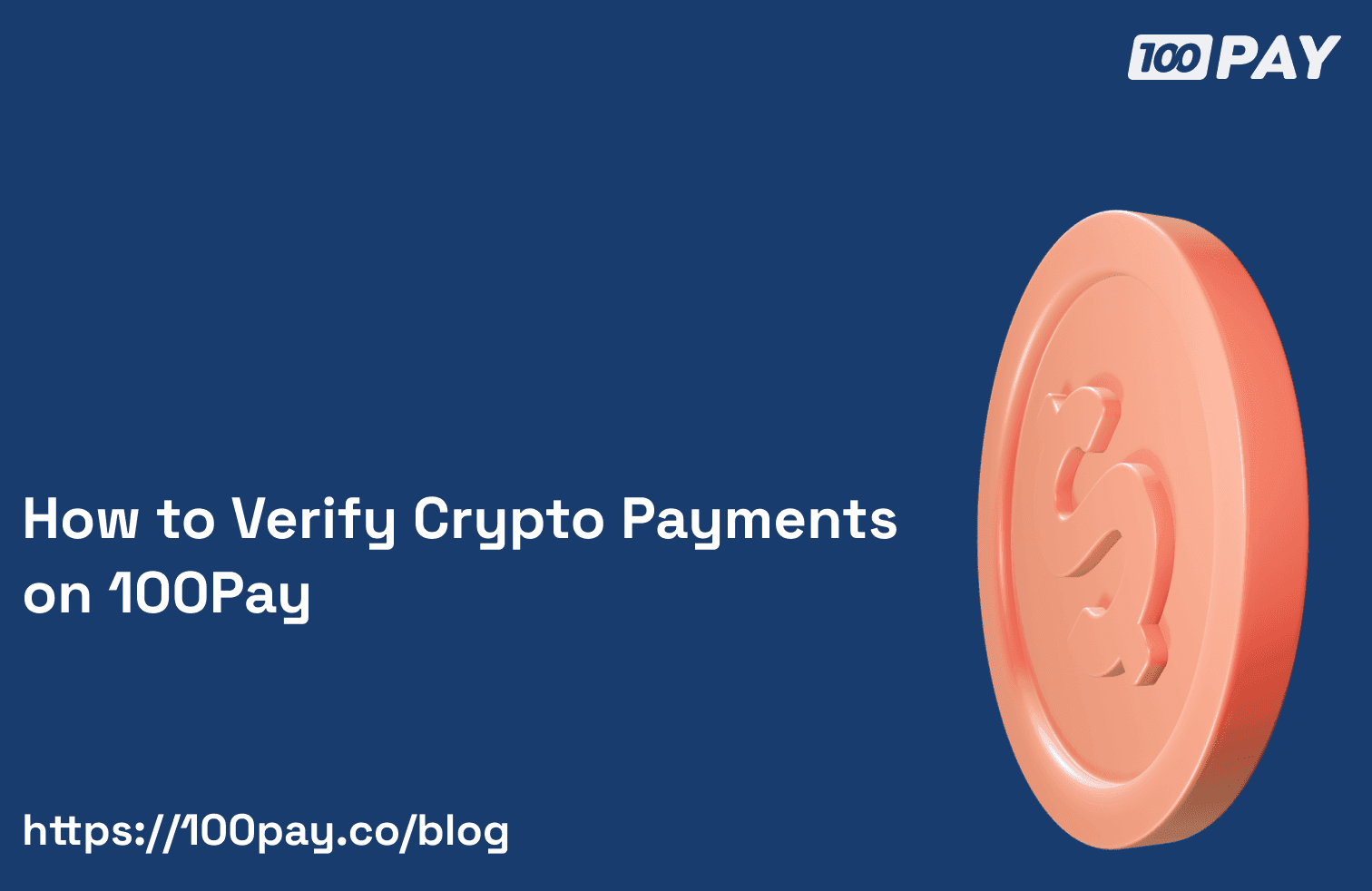
How to verify crypto payments on 100pay
In this article, I will walk you through verifying crypto payments on 100Pay before giving users value.
Let's start with a sample code:
Example Nodejs Implementation
First run npm install @100pay-hq/100pay.js
to install the 100Pay API wrapper SDK "100pay.js":
import { _100Pay } from "@100pay-hq/100pay.js";
const verifyCryptoPayment = async (chargeId) => {
return new Promise( async (resolve, reject) => {
const _100pay = new _100Pay(process.env._100PAY_PUB_KEY, process.env._100PAY_SEC_KEY)
const response = await _100pay.verify(chargeId)
// console.log('verified data ====>', { response })
if(response.status === 'error') {
// an error occurred, do something
// return res.status(400).end()
return console.log('an error occurred');
}
const chargeStatus = response.data.data.charge.status.value;
if(chargeStatus === 'paid' && response.data.data.charge.billing.currency === store.currency.currency){
// payment received, give the merchant value
console.log('paid')
updateOrderStatus(response.data.data.charge.status.total_paid)
}
if(chargeStatus === 'underpaid'){
// customer made a partial payment, send an email to the customer to complete the payment.
console.log(' ==> underpaid')
}
if(chargeStatus === 'overpaid'){
// customer paid more than what was expected, notify the customer for a balance refund via 100Pay.
console.log('===> overpaid')
let newAmount = +response.data.data.charge.status.total_paid - +response.data.data.charge.billing.amount
updateOrderStatus(newAmount)
// send customer an email for a balance refund
}
})
}
Please Note: the above code is for a nodejs environment, do not run on the client side to avoid exposing your private and secret keys.
Example php code
The PHP code below uses the cURL library to make HTTP requests and process the response:
<?php
function verifyCryptoPayment($chargeId) {
$publicKey = 'your_public_key';
$secretKey = 'your_secret_key';
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://api.100pay.co/api/v1/pay/crypto/payment/$chargeId");
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "POST");
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode(["charge_id" => $chargeId]));
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'api-key ' . $secretKey
]);
$response = curl_exec($ch);
curl_close($ch);
$responseData = json_decode($response, true);
if ($responseData['status'] === 'error') {
// an error occurred, do something
echo 'An error occurred';
return;
}
$chargeStatus = $responseData['data']['charge']['status']['value'];
if ($chargeStatus === 'paid' && $responseData['data']['charge']['billing']['currency'] === $storeCurrency) {
// payment received, give the merchant value
echo 'Paid';
updateOrderStatus($responseData['data']['charge']['status']['total_paid']);
}
if ($chargeStatus === 'underpaid') {
// customer made a partial payment, send an email to the customer to complete the payment.
echo 'Underpaid';
}
if ($chargeStatus === 'overpaid') {
// customer paid more than what was expected, notify the customer for a balance refund via 100Pay.
echo 'Overpaid';
$newAmount = floatval($responseData['data']['charge']['status']['total_paid']) - floatval($responseData['data']['charge']['billing']['amount']);
updateOrderStatus($newAmount);
// send customer an email for a balance refund
}
}
function updateOrderStatus($amount) {
// Implement the logic to update the order status with the given amount
}
$chargeId = 'your_charge_id'; // Replace with the actual charge ID
verifyCryptoPayment($chargeId);
?>
In this PHP code:
- Replace
'your_public_key'
and'your_secret_key'
with your actual public and secret keys. - Adjust the logic in the
updateOrderStatus
function to update the order status as needed. - Replace
'your_charge_id'
with the actual charge ID you want to verify.
Please note that this example uses cURL for making the API request and processing the response. Make sure to adjust the code according to your specific needs and the libraries available in your PHP environment.
Create an endpoint in your backend and call it verify-crypto-payment
. copy and paste the above code on your backend. Call this endpoint whenever the onPayment
callback function in the 100Pay checkout charge object is trigered on your frontend. You should do this to ensure a malicious user does not trick you to give value for an unpaid transaction.
Cheers 🍻